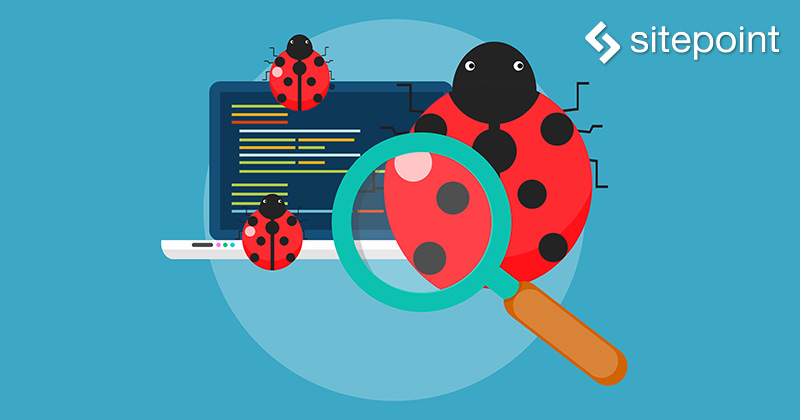
FabricはTwitterが提供するアプリ開発支援ツールです(訳注:2017年1月、TwitterはグーグルにFabricを売却しました)。この記事では、Androidアプリを例に、クラッシュの検知に役立つクラッシュレポーティング機能の説明をします。
Fabricプラグインの追加
Android Studioで新規プロジェクトを作成し、ミニマムAPIレベルを18に設定して、空のActivityを追加してください。
Android Studio plugin managerを開き、Browse Repositoriesをクリックして、Fabricを探してインストールします。プラグインを有効化するために、Android Studioを再起動すると、ツールバーに新しいアイコンが表示されます。
アイコンをクリックするとAndroid Studioの右側のウィンドウにパネルが開きます。パワーボタンをクリックしてFabricにログインもしくは新規登録し、NextをクリックするとCrashlyticsがインストールされます。
「Fabricがアプリケーションに加える変更を表示します」を確認して画面右下のApplyをクリックします。
強制的にクラッシュを発生させる
下のコードは、アプリ内のボタンをクリックすると強制クラッシュするアプリのサンプルです。
activity_main.xmlを開いて、必要のない「Floating Action Bar Button」のコードを削除します。
content_main.xmlの中に、Crashlyticsがサポートするレポートタイプに該当する2つのボタンを追加します。
TextViewを以下のコードで置き換えます。
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="50dp">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Simple Crash"
android:onClick="forceCrash"
android:layout_gravity="center" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Extra Info Crash"
android:onClick="showUserDialog"
android:layout_gravity="center"
android:layout_marginTop="30dp" />
</LinearLayout>
MainActivity.javaクラスを開いて「Floating Action Button」のコードを削除します。onCreate()の前に以下を追加します。
private EditText userIdentifierEditText, userEmailEditText, userNameEditText;
private String userIdentifier, userEmail, userName;
これらの変数は、カスタムユーザー情報を含む2つ目のレポートタイプで使われます。
1つ目のクラッシュはRuntimeExceptionをスローして、クラッシュを発生させます。
onCreate()の後ろに以下を追加します。
public void forceCrash(View view) {
throw new RuntimeException("This is a simple crash");
}
ボタンがクリックされてviewが呼ばれると、関数はViewを変数として受け取り、publicを呼び出します。forceCrashがRuntimeExceptionを投入して、Fabricのダッシュボードにクラッシュレポートを送信します。レポートには、クラッシュが発生したコードと、一般的なデバイスの情報が含まれます。
ユーザー情報を含んだクラッシュを発生させる
2つ目のボタンは、ユーザーがダイアログに入力した情報を含んだクラッシュを発生させます。たとえば、ユーザーのEメールやソーシャルネットワークのユーザーネームです。
forceCrash(View view){}の後ろに以下の関数を追加します。
public void logUser(String userIdentifier, String userEmail, String userName) {
// TODO: Use the current user's information
// You can call any combination of these three methods
Crashlytics.setUserIdentifier(userIdentifier);
Crashlytics.setUserEmail(userEmail);
Crashlytics.setUserName(userName);
}
この関数はString型の3つのパラメーターを必要とし、レポートに追加します。データを取得するために、3つのインプットフィールドを含むカスタムダイアログが必要になります。
custom_dialog_layout.xmlを作成して、以下のコードを追加します。
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/userIdentifier"
android:inputType="number"
android:hint="User Identifier:"/>
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/userEmail"
android:hint="User Email:"/>
</android.support.design.widget.TextInputLayout>
<android.support.design.widget.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/userName"
android:hint="User Name:"/>
</android.support.design.widget.TextInputLayout>
</LinearLayout>
MainActivity.javaに以下のメソッドを追加します。ダイアログを作成し、ユーザー情報を取得し、クラッシュを発生させて、レポートを送信します。
public void showUserDialog(View view) {
LayoutInflater inflater = getLayoutInflater();
View alertLayout = inflater.inflate(R.layout.custom_dialog_layout, null);
userIdentifierEditText = (EditText) alertLayout.findViewById(R.id.userIdentifier);
userEmailEditText = (EditText) alertLayout.findViewById(R.id.userEmail);
userNameEditText = (EditText) alertLayout.findViewById(R.id.userName);
AlertDialog.Builder alert = new AlertDialog.Builder(this);
alert.setTitle("Information");
alert.setView(alertLayout);
alert.setCancelable(false);
alert.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
alert.setPositiveButton("Crash & Send", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// code for matching password
userIdentifier = userIdentifierEditText.getText().toString();
userEmail = userEmailEditText.getText().toString();
userName = userNameEditText.getText().toString();
if (!userIdentifier.equals("") && !userEmail.equals("") && !userName.equals("")) {
logUser(userIdentifier, userEmail, userName);
Toast.makeText(getBaseContext(), "Sending extra information...", Toast.LENGTH_SHORT).show();
throw new RuntimeException("This is a crash with extra information");
}
Toast.makeText(getBaseContext(), "Information not send! Please complete all fields...", Toast.LENGTH_SHORT).show();
}
});
AlertDialog dialog = alert.create();
dialog.show();
}
2つ目のボタンがクリックされると、アプリケーションはダイアログを表示してユーザーに入力を求めます。Crash & Sendをクリックすると、フィールドに値が入っているかどうかをチェックして、クラッシュを発生させ、ユーザー情報を含んだレポートを送信します。
Crashlyticsのダッシュボードの見方
クラッシュレポートを見るために、Crashlyticsのダッシュボードのアプリ個別画面を開きます。
下のグラフは、特定のコードに対応するクラッシュです。クラッシュの詳細な情報を見るために、リスト内のアイテムをクリックします。
レポートを送信したユーザーについての詳しい情報を見るためには「Viewing latest crash (More details…)」をクリックします。
Answersの見方
Fabricは、アプリケーションのユーザー情報やイベント、動きをトラッキングするためのシンプルなツールも提供しています。
メソッド内に以下のコードを追加するだけで、Activityやユーザーが訪れたViewをトラッキングできます。
//New Answers data
Answers.getInstance().logContentView(new ContentViewEvent()
.putContentName("MainActivity")
.putContentType("View")
.putContentId("1234")
.putCustomAttribute("Custom Number", 20)
.putCustomAttribute("Screen Orientation", "Landscape"));
Answersのカスタムイベントも以下で作成できます。
Answers.getInstance().logCustom(new CustomEvent("User registered")
.putCustomAttribute("Custom Attribute", "Sample")
.putCustomAttribute("Custom", 350));
Answersは、以下のようなアプリケーションが含むさまざまなイベントに関するオプションを提供しています。
最後に
FabricのコンポーネントCrashlyticsは、クラッシュレポートを簡単に追加でき、ユーザーが遭遇する問題の解決に役立ち、アプリを改善できます。
※この記事は2017年1月18日にアップデートされました。CrashlyticsのAnswersの部分を追加しました。
(原文:Crash Reporting an Android App with Crashlytics and Fabric)
[翻訳:萩原伸悟/編集:Livit]
